Selecting the spawn point at runtime, and optimizations along the way
Spawn location selection is filled with a whole bunch of choices, and I’ll go through that in order of roughly volume-scale down to point scale. I’ll also describe some of the optimizations that were done along the way to keep the cost down as much as possible, as well as some of the filtering done at runtime based on the gameplay situation at hand.
At the top, let’s start with volume combining. Even with volumes filling a bunch of space, we didn’t necessarily want to have an entire region’s worth of volumes up at once. It didn’t make sense to be filtering out points that were hundreds of yards from the player. At the same time, breaking up the volumes meant we were duplicating things like spawn list validation checks for no reason. This was fixed with two main systems – sublevel streaming and volume collections.
Sublevel streaming is pretty standard in Unreal, so I won’t go over it in detail. For Maneater, spawn data was broken into its own sublevels with its own streaming settings so that we generally only had two or three sublevels of spawn data active at once – whatever one the player was actively in, plus the one they’re generally close to and moving in the direction of, and maybe some higher priority gameplay spaces that were always loaded. As the sublevels activated, volumes were added to our active list to be filtered and considered for active spawning further down the process.
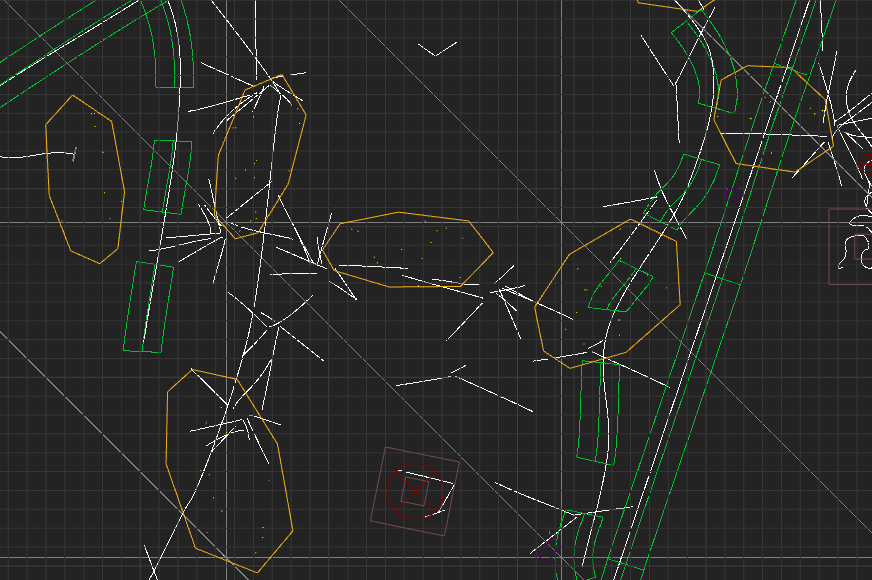
At the same time, repeating validity checks for volumes with common settings was a waste. However, we wanted to preserve flexibility for where volumes were placed in cases where it was needed. In the example above, we wanted groupers to spawn in areas roughly under garbage patches in this specific zone. This meant having volumes that, while still relatively large compared to a player, were independent in placement. By combining these at runtime, we are able to take the set of volumes that are active via sublevel streaming, and dynamically add them into a shared volume collection, combining their spawn list validation into a single pass for the entire collection, then move on to further checks from there.
With the volume collections built, I can get to the first layer of rejection – spawn conditions. Spawn lists aren’t really sufficient on their own as a way to figure out what kind of things we want to spawn. There’s also gameplay reasons behind potentially rejecting a type of spawn. Maybe we only want to let humans be on the beach during the day. Maybe a certain type of wildlife can only spawn after hitting a progression point in the story. I wrapped that all into a simple class called a spawn list condition.
UCLASS(BlueprintType, Blueprintable, Abstract) class SpawnlistCondition : public UObject { GENERATED_BODY() public: // Implementable event for native or BP. The return value of this determines // whether or not the spawn list that this is tied to can be active. UFUNCTION(BlueprintNativeEvent, Category="Condition") bool ConditionIsValid(AActor* WorldContextActor); };
From a designer facing perspective, they can do whatever they need in Blueprint with this. From a programming perspective, it means we can implement the conditions in native C++, as well as have a quick path to port things down from BP to native for performance. On the spawn end of things, it gives me a true/false way to trivially reject entire spawn lists, and if we end up with no valid spawn lists I can reject the entire volume collection from the more expensive filtering pass.
So now that I have a valid set of lists of things that can be spawned, I need to figure out where they are allowed to spawn. For that I do a quick scoring of each point to figure out if it’s valid. The scoring that I ended up using gives me a rough validity arc like this in front of the player.
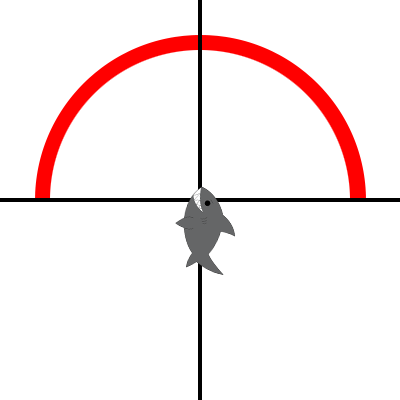
So there’s a few things to note here:
- I know it seems really weird that nothing behind the player is valid. I did a lot of testing on this. Ultimately our players were moving forward in almost all cases. On top of that, increasing spawn density in front of the player further reinforced that movement.
- Spawn cycling happens very rapidly when the player does a hard rotation, so the lack of spawning in the distance behind the player is mitigated by rapid new spawns on top of existing spawns that the player has been passing by.
- There isn’t really much in the way of blockers to guarantee we can spawn things close out of sight. Some zones have vegetation like kelp, but they also have wide open vision at or near the water’s surface. Because of this, it became more of a priority of spawning things just out of potential vision range, but anywhere. This became a bit of a happy accident in terms of greatly simplifying the selection process.
- The spawn band really is as narrow as it seems. I limited this to one spawn per frame for performance, but that’s still a whole bunch of possible spawns per second, so a wide spawn band wasn’t required to fill the scene as the player moved forward.
From a performance perspective, I was also able to go with a fairly quick approach under the mantra of “keep it simple”.
- Filtering was done on the game thread just to avoid having to deal with thread concurrency problems. We simply didn’t need to do that extra work for a small bit of gain. This became even more obvious a choice given the thread limitations of the switch (3 core CPU, no sort of hyperthreading-like tech) where simply managing threads has the potential to introduce a crippling amount of overhead.
- Each volume collection has its own delay for when it can be filtered vs using cached results. This ended up being around half a second, with a bit of randomization to enforce filter staggering. This minimized frames where large amount of volumes were being filtered at one time. Because the status of a collection doesn’t really change that much frame to frame, we could get away with a lot of delay here using old info.
- The per-point validity checks were pretty simple math (distance squared, dot product, and some multiplications for stat-based modifiers) so even with potentially thousands of points in a bad frame, we weren’t spending significant time per-point.
- There’s only a couple of types of things that actually do additional work to minimize spawning on top of each other – namely ambient beachgoers and boats.
- In the case of beachgoers, I simply put the specific spawn location on cooldown until the thing despawns or gets killed. It tracks which location it spawned at, and shoots a callback to the volume when it goes away to place the point back into the active list.
- Boats are a bit more obvious when they spawn on top of each other, so for those I do some additional range checks to minimize the likelihood of them spawning on top of each other, but it’s not 100%. Going 100% prohibitive wasn’t worth the performance cost given the low chance of the edge cases occurring.
- Otherwise, things spawn on top of each other all the time and it’s just not noticed. As an example, fish typically spawn about 50-100% further than your max viewing distance, so by the time you get to them it’s a pretty low chance that even if the same spawn location was selected twice, that the fish won’t have swam in different enough directions to no longer be overlapping. The performance cost of preventing overlaps simply wasn’t worth it.
- Each level of filtering was a significant performance improvement. Going to streamed sublevels meant a huge reduction in possible points checked each frame. Spawn list condition rejections meant a huge reduction in points that have nothing to spawn at the current game time. Spawn volume collections meant greatly reducing overlap of spawn list condition checks, particularly expensive BP conditions. Adding a filtering delay and using cached information meant that I might only be checking a single volume collection every few frames for new data, rather than all collections every frame.
- All told, I ended up getting spawn list condition rejection down to a goal of about 0.25ms and per-frame point filtering costs down to a goal of about another 0.25ms per frame, so doing additional heavy work to thread that out wasn’t worth further effort.